JSON2Video API offers a variety of export options to choose from, including publishing to FTP or SFTP servers. This means that you can easily automate the upload your videos to their final destination without checking continously the render status.
In addition to these export options, the API also allows you to define a call a webhook once the export process has finished. This feature can be incredibly useful for tracking the progress of your video exports or triggering additional actions once the export is complete.
exports property
The exports
property of the movie
object is an array used to configure the export process, with the following options:
{
"resolution": "full-hd",
"quality": "high",
"scenes": [],
"exports": [{
"destinations": [{
"id": "my-destination-id",
}]
}]
}
Currently, the exports
array only supports one item, but the destinations
array can be used to specify multiple destinations.
destinations property
The destinations
property of the exports
object is an array used to configure the different destinations of the export process.
You can specify multiple destinations for example to publish to more than one SFTP server.
Each destination item is an object with different properties depending on the type of destination.
Destination items are executed in the order they are specified in the destinations
array, therefore
the first destination item in the destinations
array will be executed first,
the second item will be executed after the first one finishes, etc.
The following types of destinations are currently supported:
The process of exporting to all the destinations
is limited to 5 minutes.
If any of the uploads takes too much time, the process may timeout and can be aborted.
Defining destinations
For secutity reasons, it's not a good idea to include connection details and credentials in
every call to the API, as they can be accidentally exposed.
A solution to this is to use the Connections in the dashboard to store all or part of the destination details
backend side and refer to it by an id
.
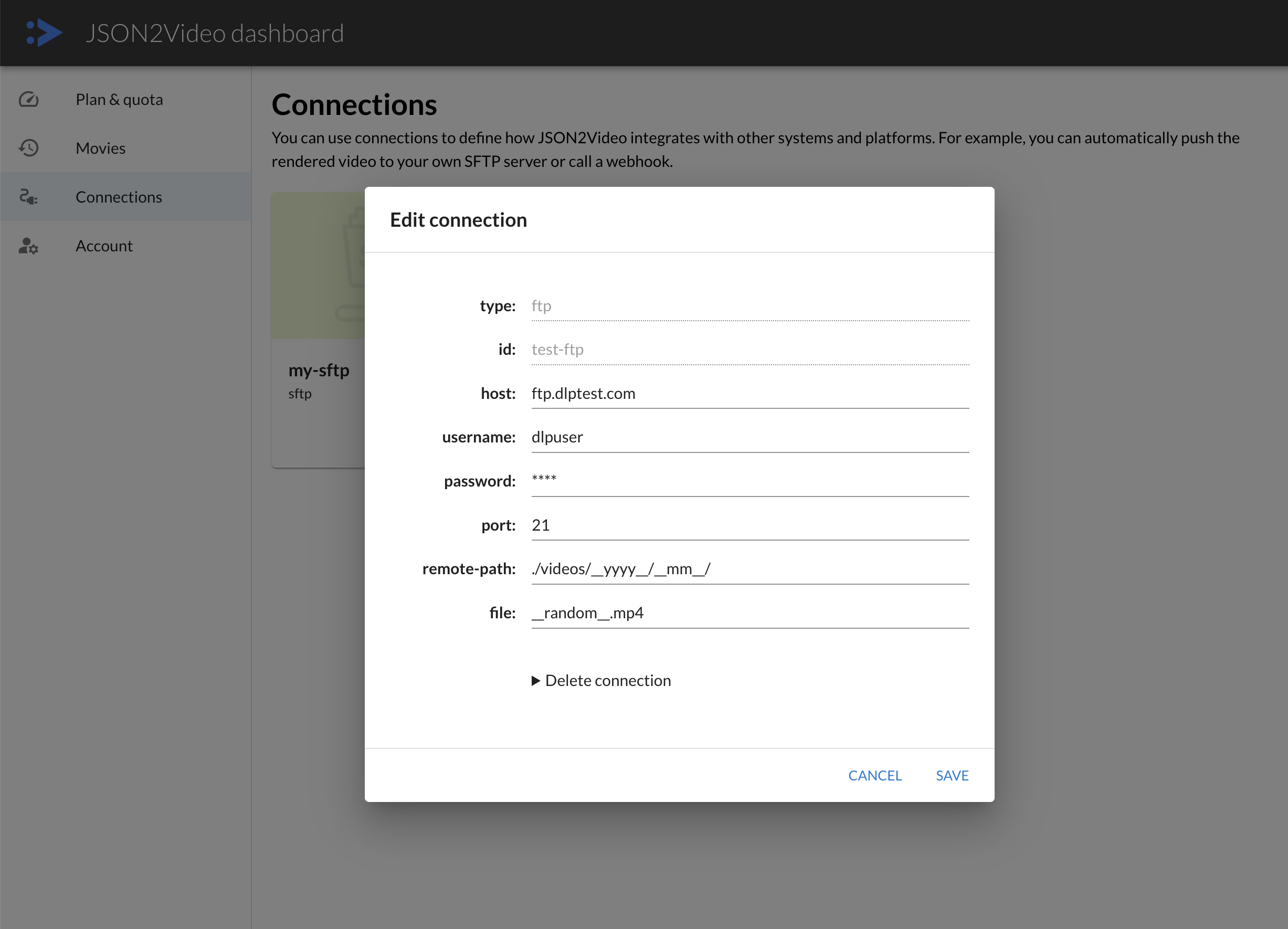
The id
is used to refer to the connection in the Connections section of the dashboard.
In the example above, the id
is test-ftp
and the JSON would look like this:
{
"resolution": "full-hd",
"quality": "high",
"scenes": [],
"exports": [{
"destinations": [{
"id": "test-ftp"
}]
}]
}
Sensible fields (like passwords or API keys) are encrypted on our side to keep them safe.
If needed, any of the properties (with the exception of type
) can be overwritten real-time in the destination
object, for example:
{
"resolution": "full-hd",
"quality": "high",
"scenes": [],
"exports": [{
"destinations": [{
"id": "test-ftp",
"file": "myfile.mp4"
}]
}]
}
macros
Fields values can include macros that are evaluated at runtime and can be used to generate dynamic values.
This is specially usefull when you want to set the name of the file that will be uploaded to a server, or create an email message that includes the URL to download the file.
- __yyyy__: the year number (example: 2023)
- __mm__: the month number (example: 12)
- __dd__: the day number (example: 25)
- __hh__: the hour number (example: 12)
- __nn__: the minute number (example: 30)
- __ss__: the second number (example: 00)
- __random__: a random number (example: 31648)
- __filename__: the original file name created by the API (example: 2023-03-25-38267.mp4)
- __filename_without_extension__: the original file name without extension (example: 2023-03-25-38267)
- __filename_extension__: the original file name without extension (example: mp4)
Example
Here is an example of using macros:
{
"resolution": "full-hd",
"quality": "high",
"scenes": [],
"exports": [{
"destinations": [{
"id": "my-ftp",
"remote-path": "./videos/__yyyy__/__mm__/",
"file": "__random__.mp4"
}]
}]
}
SFTP and FTP destinations
FTP and SFTP destinations require providing the following properties:
- type: ftp | sftp
- host: this can be an IP or a domain
- port: 21, 22 or any other port you are using
- username
- password
And optionally:
- remote-path: the remote folder where the movie will be uploaded. If not provided, it defaults to
./
- file: the file name the movie will be renamed to. If not provided, it gives a unique name based on date and a random number
The remote-path
and the file
properties can include dynamic macros that are evaluated at runtime.
The available macros are:
Example
Here is an example of a FTP destination:
{
"resolution": "full-hd",
"quality": "high",
"scenes": [],
"exports": [{
"destinations": [{
"type": "ftp",
"host": "ftp.dlptest.com",
"port": 21,
"username": "dlpuser",
"password": "rNrKYTX9g7z3RgJRmxWuGHbeu",
"remote-path": "./videos/__yyyy__/__mm__/",
"file": "__random__.mp4"
}]
}]
}
As said, it's not recommended passing credentials in your API calls.
Better set up your credentials in the dashboard and reference to them
in the destinations
object with the id
property.
Email destinations
You can also send an email when the video render finished, for notification purposes or to trigger an action.
This is how you can easily automate the publication of videos to Youtube. To learn more, read this tutorial: Publishing your videos to Youtube automatically
Email destinations require the following properties:
- type: email
- to: email address
- subject: subject of the email message
- message: body of the email message
You can use macros in the property values to generate dynamic values.